Quickstart
Start building on Basic in minutes.
Create your project
Create your project
After creating your account, head to the Dashboard and create a new project using the button on the top right.
Note your project ID, database URL, and API key (keep this a secret!)
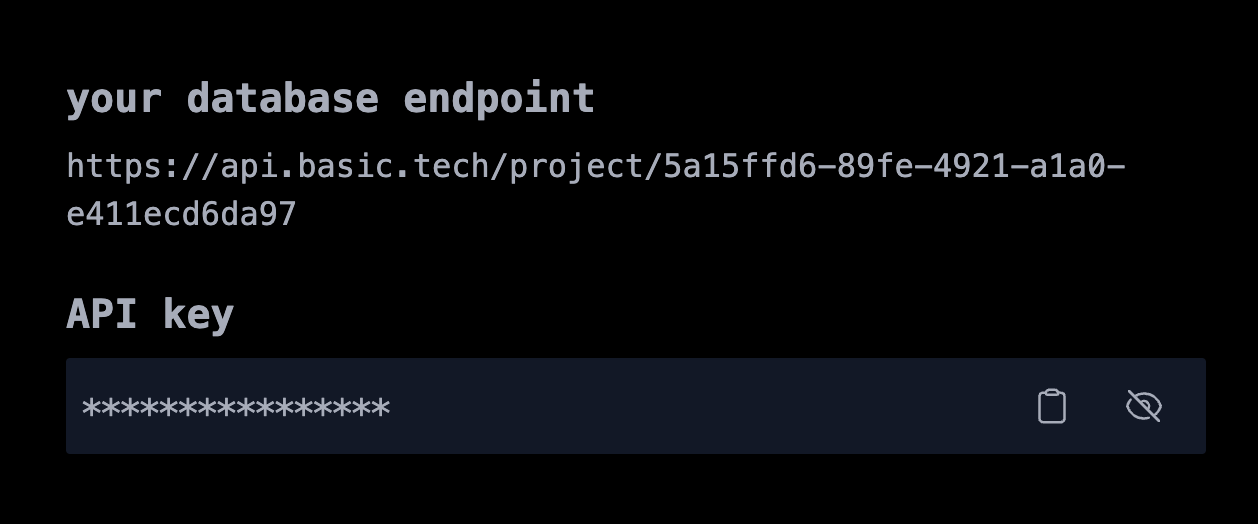
You can test everything is working by making a sample fetch request to your database:
Make sure to replace <YOUR_PROJECT_ID>
and <YOUR_API_KEY>
.
fetch("https://api.basic.tech/project/<YOUR_PROJECT_ID>", {
headers: {
"Authorization": "Bearer <YOUR_API_KEY>",
},
}).then(response => response.json())
.then(data => console.log(data));
Create your database
Go the Schema tab, and create a new table.
You can now query all the documents in the table
fetch("https://api.basic.tech/project/<YOUR_PROJECT_ID>/db/<ACCOUNT_ID>/<TABLE_NAME>", {
headers: {
"Authorization": "Bearer <YOUR_API_KEY>",
},
}).then(response => response.json())
.then(data => console.log(data));
Since we don’t have any documents created yet, this should return an empty array.
For the account_id
parameter, you can use “self”.
Add your first document
fetch("https://api.basic.tech/project/<YOUR_PROJECT_ID>/db/<ACCOUNT_ID>/<TABLE_NAME>", {
method: "POST",
headers: {
"Authorization": "Bearer <YOUR_API_KEY>",
},
body: JSON.stringify({
"hello": "world"
})
}).then(response => response.json())
.then(data => console.log(data));
If we retry the query from step 2, you should now see your document!
Update the document
Let’s update the document now. We’ll provide a document ID, and some additional data we want to store. By default, this will merge the data, instead of replacing it.
fetch("https://api.basic.tech/project/<YOUR_PROJECT_ID>/db/<ACCOUNT_ID>/<TABLE_NAME>", {
method: "POST",
headers: {
"Authorization": "Bearer <YOUR_API_KEY>",
},
body: JSON.stringify({
"sup": "universe"
})
}).then(response => response.json())
.then(data => console.log(data));
Next steps
You can now start building your app! Create tables, add items, and query to your hearts content.
In this limited preview, you are only able to store data for your own account. Support for adding additional users to your app coming soon.